Shell scripting is a powerful way to automate tasks and streamline your work on Unix-based systems (like Linux and macOS). By writing shell scripts, you can instruct the operating system to execute a series of commands. Shell scripts are especially helpful for repetitive tasks, making them essential for system administrators, developers, and even casual users looking to enhance productivity.
Lets start taking about the concepts of shell scripting/bash scripting.
1. Variables
Shell variables are in uppercase characters by convention. Bash keeps a list of two types of variables
- Global variables: Global variables or environment variables are available in all shells. The env or printenv commands can be used to display environment variables.
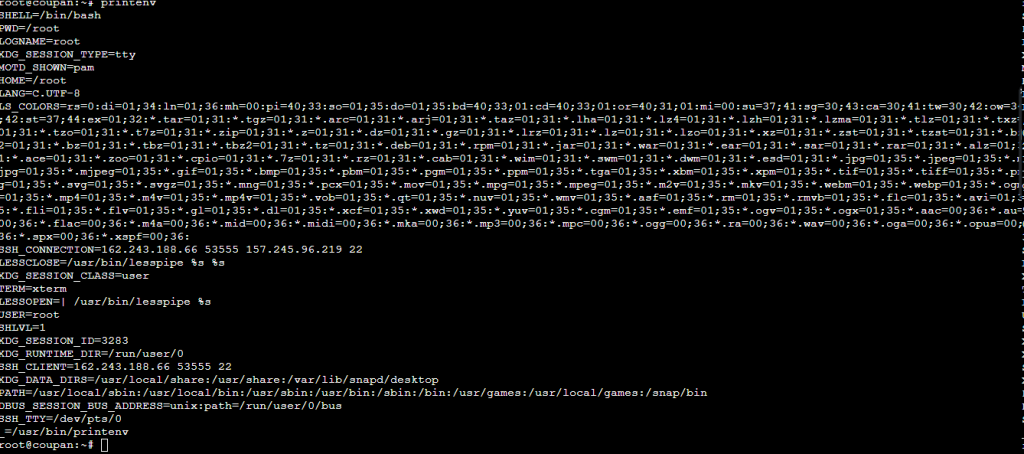
2. Local Variables: A variable created like the ones in the example above is only available to the current shell. It is a local
variable: child processes of the current shell will not be aware of this variable.
To assign a local variable you can use write like this NAME=Harry
and then to call the variable you can use echo command like this echo $NAME
.

2. Regular expressions
A regular expression is a pattern that describes a set of strings. Regular expressions are constructed analogously to arithmetic expressions by using various operators to combine smaller expressions.
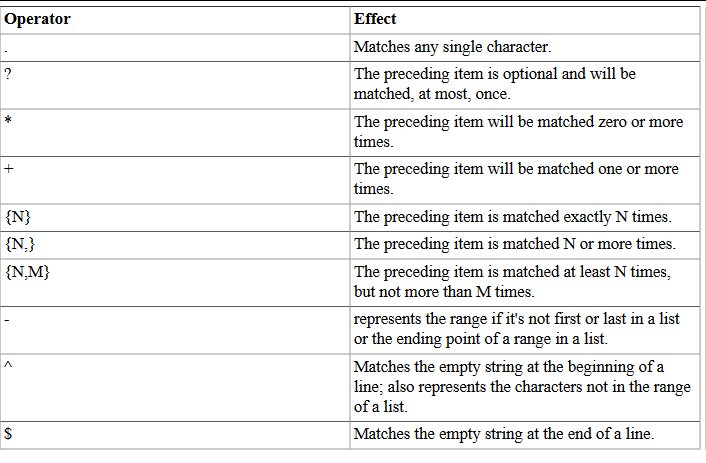
Examples using grep
You can filter out root user using this command grep ^root /etc/passwd

If you want to see only two characters in the /etc/group file you can use this command grep [yf] /etc/group
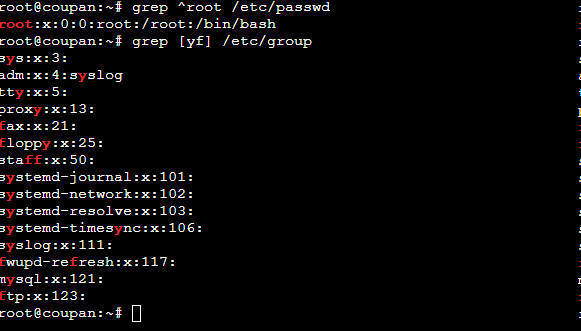
3. Conditional Statements
In Bash scripting, conditional statements allow scripts to make decisions based on specific conditions, enabling more dynamic and responsive functionality.
if
Statementif-else
Statementif-elif-else
Statement
Let’s see a example of a simple if-else statement, if-else starts with the keyword if and then we write the condition and then we end it with a semicolon in the next line we write the then keyword and after that in the next line we write the command to executed and in the next line we write the else keyword and in the next line we have to write the command executed in the else logic and we will end this with fi keyword.
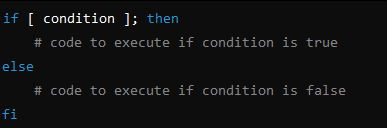
Lets see an real life example of if-else statement where we will start with declaring a variable num=10 and then we will check this condition $num -gt 5
if the variables num value is greater than 10 it will print out this statement The number is greater than 5 and in the else part we will check that if variable value is equals to 5 using this $num -eq 5
then it will print out The number is 5.
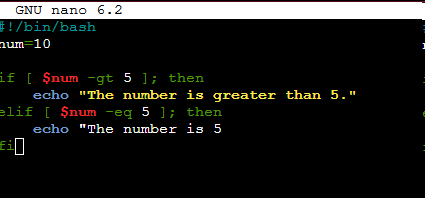
4. While loop
The while construct allows for repetitive execution of a list of commands, as long as the command controlling the while loop executes successfully (exit status of zero). The syntax is as follows:

- The
condition
inside[
and]
is evaluated before each iteration. - As long as the
condition
returns true, the commands inside the loop will be executed. - The loop stops once the
condition
evaluates to false.
Example
Here’s a simple example that prints numbers from 1 to 5:
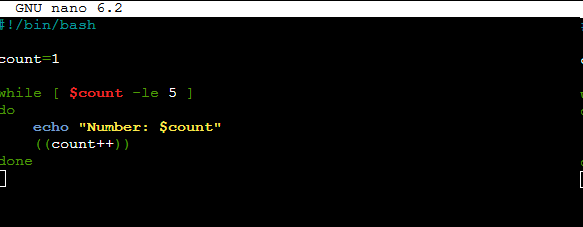
you have to also give executable permissions to the script using this command chmod +x script.sh
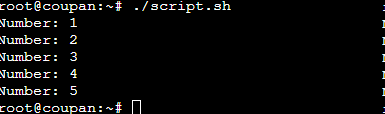
5. for Loop
The for
loop in Bash is used to iterate over a list of items or a range of numbers. It’s particularly useful for performing repetitive tasks over a known set of values, like processing files in a directory, iterating through numbers, or handling lists of strings.
Syntax
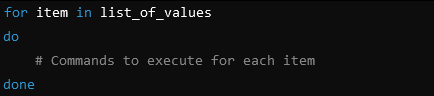
list_of_values
can be a list of strings, numbers, or filenames.- The loop iterates through each item in
list_of_values
and executes the commands within thedo ... done
block in the script.
Example
Looping Over a List of Items
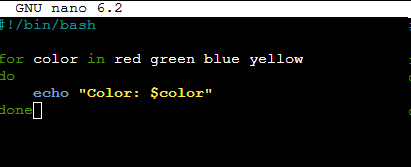
The loop iterates over the for loop and it prints out red, green, blue and yellow in the terminal.
The for
loop is very versatile and widely used for handling sequences or sets of values in scripts.
6. Case Statement
The case
statement in bash is used for conditional branching and it’s similar to if-elif-else
statements. The case
statement is particularly useful for handling multiple conditions based on a single value, like handling user input or categorizing file types. You can create scripts that accepts multiple user input using this case statements.
Syntax

variable
is the value you want to test.pattern1
,pattern2
, etc. are the possible patterns thatvariable
might match.*
is the wildcard pattern, which acts as the default if no other patterns match.;;
indicates the end of a pattern block.
Example
This is a basic example of using the case
statement to create a menu-driven program that responds to user input.
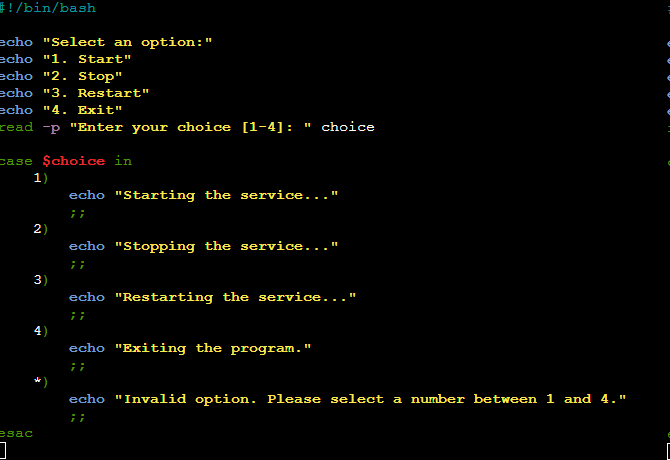
Output
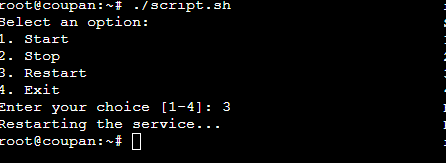
The case
statement makes it easy to handle multiple options and is commonly used for menu-driven scripts and processing user input.
7. Functions in Bash
Functions in Bash are blocks of code that can be defined once and reused multiple times throughout a script. They are useful for organizing code, avoiding repetition, and making scripts more readable and maintainable.
Defining a Function
The syntax to define a function in Bash is:
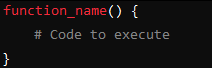
You can define a function with the function
keyword:
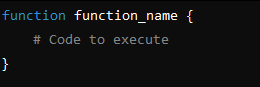
Calling a Function
To call a function, simply write its name:
function_name
Example: Function with Multiple Arguments and a Return Value
You can also use multiple arguments and a return value in a function. Here’s an example of a function that adds two numbers.
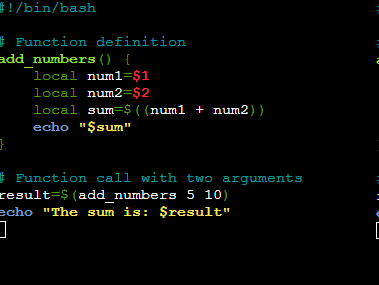
Output
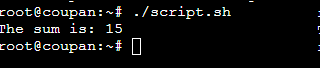
Functions in Bash allow you to reuse code and make scripts modular.
Conculsion
In conclusion, shell scripting is an essential tool for automating tasks on Unix-based systems like Linux and macOS. This beginner’s guide provides foundational knowledge of shell scripting. Each of these elements allows users to write efficient, reusable, and modular scripts that can streamline workflows and increase productivity. Whether you’re a system administrator, developer, or a casual user, these basics will enable you to handle repetitive tasks with ease and unlock the full potential of shell scripting for your work.